How to create new fields and use them¶
Three main types of field exist in axel software : spatial fields, parametric fields and discrete fields. The goal of the following text is to help you to determine which kind of field you need to use or create for your work and how you could simply use your field.
The more general type of the field hierarchy in axel is axlAbstractField
. Then there are three main abstract classes which represent the three types of field mentionned above, respectively : axlAbstractFieldSpatial
, axlAbstractFieldParametric
and axlAbstractFieldDiscrete
.
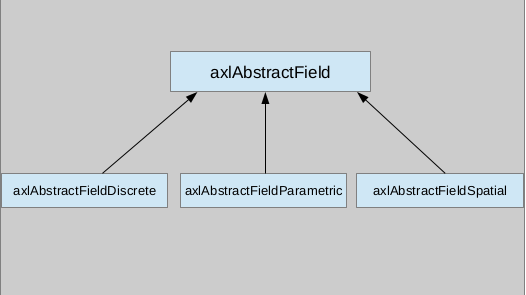
What kind of field should I use ?¶
Spatial field¶
This field type is characterized as follow : a function is given, f(x,y,z), that returns a value (scalar, vector or tensor) which depends on the 3D point on which the field is evaluated. It could be applied on any Axl data. The only constraint is the existence of the function at every points of the evaluation domain. Every spatial field must inherit from axlAbstractFieldSpatial
which determines the main methods that you have to implement for your spatial field. An example is axlFieldSpatialCoordinate.
Parametric field¶
This type of field can be applied on parametrized data, for instance BSpline data in Axl. Like spatial field it is evaluated thanks to a function which returns a value. But in this case this value depends on the parameters values. The methods which are necessary to be implemented in your parametric field are given by axlAbstractFieldParametric
. An example is axlFieldParametricSurfaceTangentVector.
Discrete field¶
This last axel field type is inspired by the VTK fields. The value of a discrete field are stored in an vtkDataArray which size equals to the number of elements of the mesh support. This kind of field is useful when you don’t have access to the function which computes the field values but only to some discrete results. The field is calculated only on the mesh nodes of the object it is applied on. The implementation structure of this kind of field is given by axlAbstractDiscreteField
. An example is axlFieldDiscrete.
List of fields that already exist¶
Some fields are already implemented in Axl software such as:
axlFieldSpatialCoordinates
axlFieldSpatialPointDistance
axlFieldDiscrete
axlFieldParametricCurveTangentVector
and so on.
You can see them all on the axel documentation of axlAbstractField. Check that the field you need for your work doesn’t exist in Axl or in one of its available plugins.
How to create my process field creator ?¶
Now you know what field you must use. We supposed in this part that you have created your own field, as previously described, if it didn’t exist already in Axl software. Once the field object exists, you need to provide a process which will create one (or several) fields. Here are some methods you need to use to create your field, illustrated by a process creator example:
int axlFieldSpatialCoordinatesCreator::update(void)
{
if(!d->input)
return 0;
//First Step : create field
// use field constructor
d->spatialField = new axlFieldSpatialCoordinates();
// check if the name given in the constructor, by default the identifier,
// is already used.
QString newName = d->input->changeFieldName(d->spatialField->objectName());
// give a name to the field.By default the identifier,
//otherwise the identifier followed by the number of occurence of the same
//field type applied on the parent object.
d->spatialField->setObjectName(newName);
// add the field created to the list of field applied on the input of the process.
//This input become the parent object of the new field.
d->input->addField(d->spatialField);
if(!d->spatialField) // field not found !!
return 0;
//Second step : fill some parameter values of the field.
// (only if your field has parameters, optional)
d->spatialField->setParameter(d->a,0);
d->spatialField->setParameter(d->b,1);
d->spatialField->setParameter(d->c,2);
// Last Step :
// say that your field has been updated/Modified
d->spatialField->update();
// send it to the axlView using signal dataSetFieldsChanged.
QList<axlAbstractData *>list;
list << d->input;
emit dataSetFieldsChanged(list, d->spatialField->objectName());
return 1;
}
As shown in this example there are three main steps you have to follow to create your field :
- First step is to create your field and to give it a name which is not used by another field applied on the parent object. This is made by using the
axlAbstractData
methodchangeFieldName(QString fieldName)
. This method is used on the input of the process field creator to check if the default name given by the constructor of the field is not used for any other field applied on the input. By default the field name is equal to the field identifier. For instance if the field is anaxlFieldSpatialCoordinates
then its default name will beaxlFieldSpatialCoordinates
. If a field has already this name thenchangeFieldName
will return a new name for the new field. This name will composed of the identifier on which will be appended the number of occurence of the field of same type applied on the input. For example if the new field is aaxlFieldSpatialCoordinates
and a field with this name exists thenchangeFieldName
will returnaxlFieldSpatialCoordinates1
. If a new field of this type is applied later on the input it will be calledaxlFieldSpatialCoordinates2
etc …- Second step is optional. It is useful only if your field kind has parameters. It ‘ll be also necessary if the field constructor doesn’t express some general field properties like the support etc (for instance :
field->setType(axlAbstractField::Double)
;field->setKind(axlAbstractField::Scalar)
;field->setSupport(axlAbstractField::Point)
; the array size of anaxlFieldDiscrete
).- The last step is very important. If you don’t implement it your field won’t be displayed in the view.